diff --git a/Exercises.ru.md b/Exercises.ru.md new file mode 100644 index 0000000..038ef43 --- /dev/null +++ b/Exercises.ru.md @@ -0,0 +1,47 @@ +# Упражнения + +## Итерирование циклами + +Реализуйте функцию `sum(...args)`, которая суммирует все свои аргументы, пятью +разными способами. Примеры вызовов с результатами: +```js +const a = sum(1, 2, 3) // a === 6 +const b = sum(0) // b === 0 +const c = sum() // c === 0 +const d = sum(1, -1, 1) // d === 1 +const e = sum(10, -1, -1, -1) // e === 7 +``` + +1. Цикл `for` +2. Цикл `for..of` +3. Цикл `while` +4. Цикл `do..while` +5. Метод `Array.prototype.reduce()` + +## Итерирование по двумерному массиву + +6. Найдите максимальный элемент в двумерном массиве +```js +const m = max([[1, 2, 3], [4, 5, 6], [7, 8, 9]]); +console.log(m); // 9 +``` + +## Итерирование объектов-справочников + +7. При помощи цикла `for..in` перебрать объект-справочник с датами рождения и +смерти людей и вернуть справочник с продолжительностью их жизни. Например: +```js +const persons = { + lenin: { born: 1870, died: 1924 }, + mao: { born: 1893, died: 1976 }, + gandhi: { born: 1869, died: 1948 }, + hirohito: { born: 1901, died: 1989 }, +}; +console.log(ages(persons)); +// { +// lenin: 54, +// mao: 83, +// gandhi: 79, +// hirohito: 88, +// } +``` diff --git a/Exercises/1-for.js b/Exercises/1-for.js index 62e6ab8..9c605a2 100644 --- a/Exercises/1-for.js +++ b/Exercises/1-for.js @@ -1,9 +1,12 @@ -'use strict'; +/* eslint-disable quotes */ +"use strict"; -const sum = (...args) => { - // Use for loop and accumulator variable - // to calculate sum of all given arguments - // For example sum(1, 2, 3) should return 6 +const sum = args => { + let add = 0; + for (let i = 0; i < args.length; i++) { + add += Number(args[i]); + } + return add; }; module.exports = { sum }; diff --git a/Exercises/2-for-of.js b/Exercises/2-for-of.js index 9965f25..8853e1d 100644 --- a/Exercises/2-for-of.js +++ b/Exercises/2-for-of.js @@ -1,9 +1,13 @@ -'use strict'; +/* eslint-disable quotes */ +"use strict"; const sum = (...args) => { - // Use for..of loop and accumulator variable - // to calculate sum of all given arguments - // For example sum(1, 2, 3) should return 6 + let add = 0; + for (const i of args) { + add += i; + } + return add; }; + module.exports = { sum }; diff --git a/Exercises/3-while.js b/Exercises/3-while.js index 6110b9f..1bb0485 100644 --- a/Exercises/3-while.js +++ b/Exercises/3-while.js @@ -1,9 +1,14 @@ 'use strict'; const sum = (...args) => { - // Use while loop and accumulator variable - // to calculate sum of all given arguments - // For example sum(1, 2, 3) should return 6 + let add = 0; + let index = args.length - 1; + while (index >= 0) { + add += args[index]; + args.pop(); + index--; + } + return add; }; module.exports = { sum }; diff --git a/Exercises/4-do-while.js b/Exercises/4-do-while.js index 22d4464..e542ef0 100644 --- a/Exercises/4-do-while.js +++ b/Exercises/4-do-while.js @@ -1,9 +1,14 @@ -'use strict'; +// eslint-disable-next-line quotes +"use strict"; const sum = (...args) => { - // Use do..while loop and accumulator variable - // to calculate sum of all given arguments - // For example sum(1, 2, 3) should return 6 + let add = 0; + let index = args.length - 1; + do { + add += args[index]; + index--; + } while (index >= 0); + return add; }; module.exports = { sum }; diff --git a/Exercises/5-reduce.js b/Exercises/5-reduce.js index a9cb44c..6735d45 100644 --- a/Exercises/5-reduce.js +++ b/Exercises/5-reduce.js @@ -1,8 +1,5 @@ 'use strict'; -const sum = (...args) => 0; -// Use Array.prototype.reduce method -// to calculate sum of all given arguments -// For example sum(1, 2, 3) should return 6 +const sum = (...args) => args.reduce((acc, curr) => acc + curr, 0); module.exports = { sum }; diff --git a/Exercises/6-matrix.js b/Exercises/6-matrix.js new file mode 100644 index 0000000..6dc37b5 --- /dev/null +++ b/Exercises/6-matrix.js @@ -0,0 +1,12 @@ +'use strict'; + +const max = matrix => + [] + .concat( + Array.isArray(matrix) ? + matrix.reduce((acc, cur) => acc.concat(max(cur)), []) : + matrix + ) + .sort((a, b) => b - a)[0]; + +module.exports = { max }; diff --git a/Exercises/6-matrix.test b/Exercises/6-matrix.test new file mode 100644 index 0000000..aedfec3 --- /dev/null +++ b/Exercises/6-matrix.test @@ -0,0 +1,13 @@ +({ + name: 'max', + length: [220, 300], + cases: [ + [[[10]], 10], + [[[1, 2], [3, 4], [5, 6]], 6], + [[[-1, 1], [2, -1], [-1, 0]], 2], + ], + test: max => { + const src = max.toString(); + if (!src.includes('for (')) throw new Error('Use for loop'); + } +}) diff --git a/Exercises/7-ages.js b/Exercises/7-ages.js new file mode 100644 index 0000000..1760e8b --- /dev/null +++ b/Exercises/7-ages.js @@ -0,0 +1,10 @@ +'use strict'; + +const ages = persons => { + const lifeTime = {}; + for (const key in persons) + lifeTime[key] = persons[key].died - persons[key].born; + return lifeTime; +}; + +module.exports = { ages }; diff --git a/Exercises/7-ages.test b/Exercises/7-ages.test new file mode 100644 index 0000000..9802861 --- /dev/null +++ b/Exercises/7-ages.test @@ -0,0 +1,24 @@ +({ + name: 'ages', + length: [150, 190], + cases: [ + [ + { + lenin: { born: 1870, died: 1924 }, + mao: { born: 1893, died: 1976 }, + gandhi: { born: 1869, died: 1948 }, + hirohito: { born: 1901, died: 1989 }, + }, { + lenin: 54, + mao: 83, + gandhi: 79, + hirohito: 88, + } + ] + ], + test: ages => { + const src = ages.toString(); + if (!src.includes('for (')) throw new Error('Use for..in loop'); + if (!src.includes(' in ')) throw new Error('Use for..in loop'); + } +}) diff --git a/README.md b/README.md index 005400b..669b1c4 100644 --- a/README.md +++ b/README.md @@ -1,4 +1,4 @@ # Different implementation of iterations as a code abstraction -[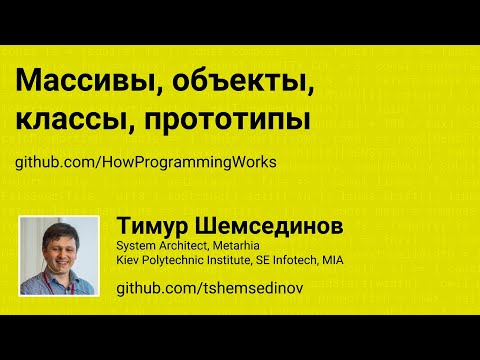](https://www.youtube.com/watch?v=/VBMGnAPfmsY) +[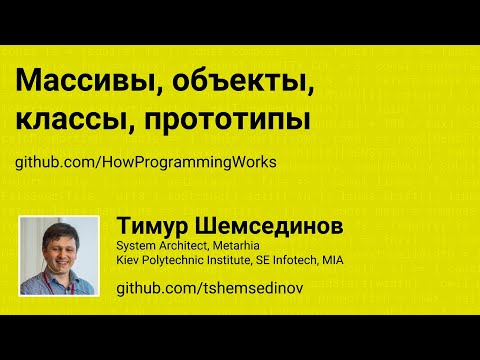](https://www.youtube.com/watch?v=VBMGnAPfmsY) [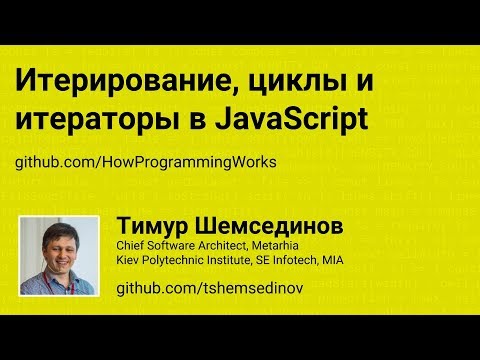](https://www.youtube.com/watch?v=lq3b5_UGJas) diff --git a/Solutions/3-while.js b/Solutions/3-while.js index 66b639c..45bcb50 100644 --- a/Solutions/3-while.js +++ b/Solutions/3-while.js @@ -3,7 +3,7 @@ const sum = (...args) => { let value = 0; while (args.length > 0) { - value += args.shift(); + value += args.pop(); } return value; }; diff --git a/Solutions/4-do-while.js b/Solutions/4-do-while.js index a2abea1..2d22dac 100644 --- a/Solutions/4-do-while.js +++ b/Solutions/4-do-while.js @@ -4,7 +4,7 @@ const sum = (...args) => { if (args.length === 0) return 0; let value = 0; do { - value += args.shift(); + value += args.pop(); } while (args.length > 0); return value; }; diff --git a/Solutions/6-matrix.js b/Solutions/6-matrix.js new file mode 100644 index 0000000..78369e8 --- /dev/null +++ b/Solutions/6-matrix.js @@ -0,0 +1,15 @@ +'use strict'; + +const max = matrix => { + let value = matrix[0][0]; + for (let i = 0; i < matrix.length; i++) { + const row = matrix[i]; + for (let j = 0; j < row.length; j++) { + const cell = row[j]; + if (value < cell) value = cell; + } + } + return value; +}; + +module.exports = { max }; diff --git a/Solutions/7-ages.js b/Solutions/7-ages.js new file mode 100644 index 0000000..ccd520f --- /dev/null +++ b/Solutions/7-ages.js @@ -0,0 +1,12 @@ +'use strict'; + +const ages = persons => { + const data = {}; + for (const name in persons) { + const person = persons[name]; + data[name] = person.died - person.born; + } + return data; +}; + +module.exports = { ages };